In our previous post, we explored the basics of deep learning and built a model to classify handwritten digits. Now, we’ll take it a step further and dive into the world of Convolutional Neural Networks (CNNs), a powerful tool in deep learning that excels at processing image data. CNNs are particularly effective at identifying patterns and features in images, making them the go-to choice for tasks like image classification, object detection, and more.
1. Introduction to Convolutional Neural Networks (CNN)
A Convolutional Neural Network (CNN) is a type of neural network designed specifically for processing grid-like data, such as images. Unlike traditional neural networks, which work well with 1D data, CNNs are optimized for 2D data like images. They automatically detect and learn features in images, such as edges, textures, and even more complex patterns.
Why do we need CNNs?
Traditional neural networks (like MLPs) treat input data as a flat array, ignoring the spatial structure of images. This is problematic because the relationship between pixels (e.g., adjacent pixels in an edge) is crucial for understanding images. CNNs, on the other hand, preserve the spatial relationships by applying convolutional operations that extract local features from the image. This makes CNNs highly effective for image-related tasks.
2. Key Components of CNNs
CNNs are composed of several types of layers, each serving a specific function. The most important layers in a CNN include:
- Convolutional Layer: This layer applies a set of filters (kernels) to the input image, creating feature maps that highlight various aspects of the image (like edges or textures).
- Pooling Layer: This layer reduces the dimensionality of the feature maps while retaining the most important information. For example, Max Pooling selects the maximum value in a region, reducing the size of the feature map.
- Activation Function: Functions like ReLU (Rectified Linear Unit) introduce non-linearity to the model, allowing it to learn complex patterns.
Let’s break down these components with a simple analogy:
Imagine you’re a detective looking at a crime scene photo. The convolutional layer acts like a magnifying glass that helps you focus on specific details (like a fingerprint). The pooling layer then helps you summarize these details so you can store them in your memory without losing important information. The activation function helps you decide which details are important enough to pass on to the next step in solving the case.
3. Building a CNN Model
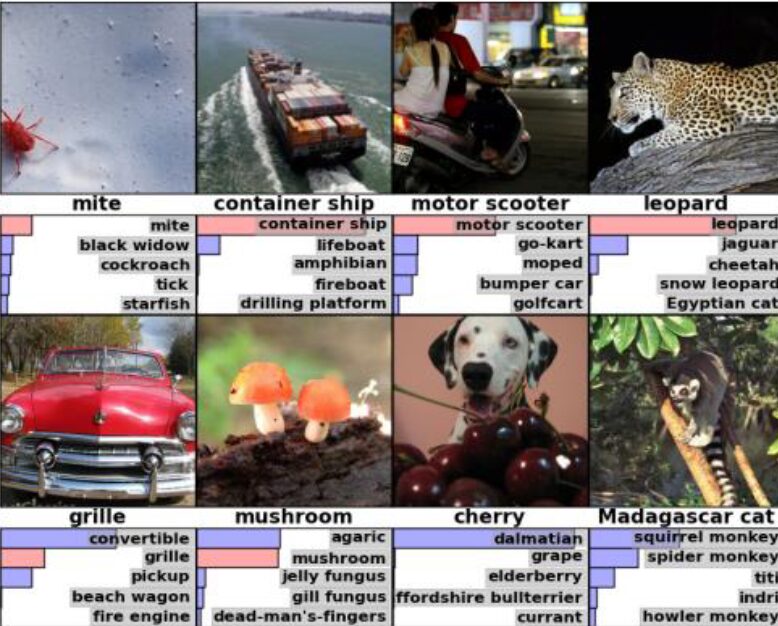
Now, let’s build a CNN model to classify images using the CIFAR-10 dataset. This dataset consists of 32×32 pixel color images across 10 different classes (e.g., airplane, car, bird, cat, etc.).
1) Loading and Preprocessing the Dataset
First, we need to load the CIFAR-10 dataset and preprocess the data. Preprocessing involves scaling the pixel values and converting class labels to a format suitable for neural networks.
from tensorflow.keras.datasets import cifar10
from tensorflow.keras.utils import to_categorical
# Load the CIFAR-10 dataset
(X_train, Y_train), (X_test, Y_test) = cifar10.load_data()
# Normalize the data (scale pixel values to the range 0-1)
X_train = X_train.astype('float32') / 255.0
X_test = X_test.astype('float32') / 255.0
# Convert labels to one-hot encoding
Y_train = to_categorical(Y_train, 10)
Y_test = to_categorical(Y_test, 10)
Key Concepts:
- Normalization: Scaling pixel values from 0-255 to 0-1 helps the model train more efficiently.
- One-Hot Encoding: Converts class labels (e.g., 0-9) into a binary vector format that the neural network can process. For example, the number 3 becomes [0, 0, 0, 1, 0, 0, 0, 0, 0, 0].
2) Building the CNN Model
We’ll use the Sequential API in Keras to build a simple CNN model. This model will consist of two convolutional-pooling blocks followed by fully connected layers.
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense
# Build the CNN model
model = Sequential()
# First convolutional-pooling layer
model.add(Conv2D(32, (3, 3), activation='relu', padding='same', input_shape=(32, 32, 3)))
model.add(MaxPooling2D((2, 2)))
# Second convolutional-pooling layer
model.add(Conv2D(64, (3, 3), activation='relu', padding='same'))
model.add(MaxPooling2D((2, 2)))
# Fully connected layers
model.add(Flatten())
model.add(Dense(128, activation='relu'))
model.add(Dense(10, activation='softmax'))
# Model summary
model.summary()
Understanding Each Layer:
- Conv2D Layer: Uses filters to extract features from the input image. Here, 32 and 64 are the number of filters, and (3, 3) is the size of each filter.
- MaxPooling2D Layer: Reduces the size of the feature maps, keeping the most important information.
- Flatten Layer: Converts the 2D feature maps into a 1D vector to be fed into the fully connected layers.
- Dense Layer: Fully connected layers that combine the features and output predictions for each class.
3) Compiling and Training the Model
Next, we compile the model using categorical crossentropy as the loss function and Adam as the optimizer. Then, we train the model.
from tensorflow.keras.optimizers import Adam
# Compile the model
model.compile(optimizer=Adam(), loss='categorical_crossentropy', metrics=['accuracy'])
# Train the model
model.fit(X_train, Y_train, epochs=15, batch_size=64, validation_data=(X_test, Y_test))
Key Concepts:
- Loss Function: Measures how well the model’s predictions match the actual labels. Categorical crossentropy is commonly used for multi-class classification.
- Optimizer: Adam is an optimization algorithm that adjusts the learning rate during training, balancing speed and performance.
- Epoch: One complete pass through the entire training dataset. We train the model over multiple epochs to improve accuracy.
4) Evaluating Model Performance
After training, we evaluate the model’s performance on the test dataset.
# Evaluate the model
test_loss, test_acc = model.evaluate(X_test, Y_test, verbose=2)
print(f'\nTest accuracy: {test_acc}')
This code calculates the model’s accuracy on the test data, helping us understand how well it generalizes to new, unseen data.
4. Enhancing Model Performance with Data Augmentation
To further improve the model’s performance, we can use Data Augmentation. This technique artificially expands the training dataset by applying random transformations to the images, such as flipping, rotating, or zooming.
from tensorflow.keras.preprocessing.image import ImageDataGenerator
# Set up the data augmentation generator
datagen = ImageDataGenerator(
rotation_range=20,
width_shift_range=0.2,
height_shift_range=0.2,
horizontal_flip=True,
)
# Apply data augmentation and train the model
datagen.fit(X_train)
model.fit(datagen.flow(X_train, Y_train, batch_size=64), epochs=15, validation_data=(X_test, Y_test))
Benefits of Data Augmentation:
- Increased Dataset Size: By generating new variations of existing images, we effectively increase the size of our training dataset.
- Improved Generalization: The model becomes more robust to variations in the input data, leading to better performance on unseen data.
5. Analyzing Errors with a Confusion Matrix
To gain deeper insights into the model’s performance, we can use a Confusion Matrix. This tool allows us to visualize how well the model distinguishes between different classes and where it might be making mistakes.
from sklearn.metrics import confusion_matrix
import seaborn as sns
import matplotlib.pyplot as plt
# Generate predictions
Y_pred = model.predict(X_test)
Y_pred_classes = Y_pred.argmax(axis=-1)
Y_true = Y_test.argmax(axis=-1)
# Create confusion matrix
cm = confusion_matrix(Y_true, Y_pred_classes)
# Visualize the confusion matrix
plt.figure(figsize=(10, 8))
sns.heatmap(cm, annot=True, fmt="d", cmap="Blues", xticklabels=['airplane', 'automobile', 'bird', 'cat', 'deer', 'dog', 'frog', 'horse', 'ship', 'truck'], yticklabels=['airplane', 'automobile', 'bird', 'cat', 'deer', 'dog', 'frog', 'horse', 'ship', 'truck'])
plt.xlabel('Predicted')
plt.ylabel('True')
plt.show()
Importance of the Confusion Matrix:
- Identifying Strengths and Weaknesses: The confusion matrix helps us identify which classes the model predicts well and which ones it struggles with.
- Spotting Patterns in Errors: We can see if the model is consistently confusing certain classes, providing insights for further model improvements.
Conclusion
In this post, we explored the power of CNNs for image
classification, walking through the process of building, training, and evaluating a CNN model. We also learned how to enhance model performance using data augmentation and how to analyze errors with a confusion matrix.
With this knowledge, you’re now equipped to tackle more complex image classification tasks using CNNs. As you practice and experiment with different architectures and datasets, you’ll gain a deeper understanding of how to optimize and deploy deep learning models in real-world applications.
In our next post, we’ll explore advanced CNN architectures and their applications in various domains. Stay tuned!
If you found this guide helpful, please subscribe for more deep learning tutorials. Feel free to leave any questions or comments below!
Related Resouce
Related Posts
- Introduction to AI and Deep Learning – CSAI
- Deep Learning Development Environment Setup Guide – CSAI
- Deep Learning in Action: Building a Handwritten Digit Classifier – CSAI